Introduction to Python Programming Language: A Comprehensive Guide with 139 Activities

Chapter 1: Getting Started with Python
1.1 What is Python?
Python is a versatile and beginner-friendly programming language that is widely used in various domains, including data science, web development, and machine learning. Its ease of learning and readability have made it popular among both beginners and experienced programmers.
4 out of 5
Language | : | English |
File size | : | 6464 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Word Wise | : | Enabled |
Print length | : | 263 pages |
Lending | : | Enabled |
1.2 Setting Up Python
- Download Python from the official website.
- Install Python on your computer.
- Verify the installation by opening a command prompt and typing "python --version".
1.3 Your First Python Program
- Open a text editor (e.g., Notepad, IDLE).
- Type the following code:
print("Hello, Python!")
- Save the file with a .py extension.
- Run the program by typing "python [file_name].py" in the command prompt.
Chapter 2: Basic Data Types
2.1 to Data Types
Python supports various data types, including strings, integers, floats, and booleans. These data types define the kind of data that a variable can hold.
2.2 String Operations
- Concatenation: '+'
- Repetition: '*'
- Slicing: [start:stop:step]
2.3 Numeric Operations
- Arithmetic operators: +, -, *, /, %
- Comparison operators: ==, !=, , =
- Boolean operators: and, or, not
Chapter 3: Variables and Operators
3.1 Variables
Variables are used to store data in Python. They must start with a letter and can contain letters, numbers, and underscores.
3.2 Operators
- Assignment operator: =
- Arithmetic operators: +, -, *, /, %
- Comparison operators: ==, !=, , =
- Logical operators: and, or, not
Chapter 4: Control Flow
4.1 Conditional Statements
- if
- else
- elif
4.2 Loop Statements
- for
- while
- break
- continue
Chapter 5: Functions
5.1 Defining Functions
Functions are reusable blocks of code that perform specific tasks. They are defined using the 'def' keyword.
5.2 Calling Functions
To use a function, simply call it by its name and pass the required arguments (inputs).
5.3 Returning Values
Functions can return values using the 'return' keyword. The returned value can be stored in a variable.
Chapter 6: Data Structures
6.1 Lists
Lists are ordered collections of elements that can be accessed using their index.
6.2 Tuples
Tuples are immutable (unchangeable) ordered collections of elements that can be accessed using their index.
6.3 Dictionaries
Dictionaries are collections of key-value pairs that can be accessed using their keys.
Chapter 7: Object-Oriented Programming
7.1 Classes and Objects
Object-oriented programming involves creating classes and objects that encapsulate data and behavior.
7.2 Inheritance
Inheritance allows classes to inherit properties and methods from other classes.
7.3 Polymorphism
Polymorphism allows objects of different classes to respond to the same method call in different ways.
Chapter 8: File Handling
8.1 Opening and Closing Files
Files are used to store and retrieve data. They are opened using the 'open()' function.
8.2 Reading and Writing Files
- Reading: 'read()'
- Writing: 'write()'
8.3 Closing Files
Files must be closed after use to release system resources.
Chapter 9: Error Handling
9.1 Exceptions
Errors and exceptions can occur during program execution. They are handled using 'try' and 'except' blocks.
9.2 Exception Types
- ValueError
- TypeError
- IndexError
9.3 Custom Exceptions
Custom exceptions can be created to handle specific errors.
Chapter 10: Modules
10.1 Importing Modules
Modules allow us to import pre-written code into our programs.
10.2 Creating Modules
We can create our own modules to organize code and promote reusability.
10.3 Module Search Path
Python searches for modules in a specific order, which can be customized.
Chapter 11: Python Projects
This chapter provides hands-on projects to apply the concepts learned throughout the guide.
This comprehensive guide has covered the essential concepts of Python programming. By completing the 139 activities, you will gain a solid foundation in Python and be equipped to tackle real-world programming challenges.
4 out of 5
Language | : | English |
File size | : | 6464 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Word Wise | : | Enabled |
Print length | : | 263 pages |
Lending | : | Enabled |
Do you want to contribute by writing guest posts on this blog?
Please contact us and send us a resume of previous articles that you have written.
Fiction
Non Fiction
Romance
Mystery
Thriller
SciFi
Fantasy
Horror
Biography
Selfhelp
Business
History
Classics
Poetry
Childrens
Young Adult
Educational
Cooking
Travel
Lifestyle
Spirituality
Health
Fitness
Technology
Science
Arts
Crafts
DIY
Gardening
Petcare
Maria Van Noord
Michael D Alessio
Cornelia Pelzer Elwood
Sherri L Jackson
Dave Rearick
Tina Schindler
Dina Nayeri
Jim Wiese
Healthfit Publishing
Pat Rigsby
Michael Gurian
Ashley Christensen
Mark Hansen
Carrie Hope Fletcher
Phil Williams
Wyatt Mcspadden
Dennis Adler
Michael Lear Hynson
The Atavist
John Jamieson
Om Krishna Uprety
L W Jacobs
Cal Newport
Leon Speroff
Shawna Richer
James Goi Jr
Jeff Belanger
Michael Tan
William Ian Miller
Marc Bona
Martha Finley
R Scott Thornton
Nikki Carroll
Lingo Mastery
David Martin
Scarlett V Clark
David Cannon
Sarah Baker
Thomas Carothers
Neville Goddard
Theodora Papatheodorou
Nawuth Keat
Zachery Knowles
Lauren Manoy
Melissa A Priblo Chapman
Lewis Kirkham
John Burroughs
Peterson S
Elizabeth Anne Wood
Leah Hazard
Joanne Kimes
Charles Salzberg
Nigel Cawthorne
Vivian Foster
Paul Rabinow
Chris Pountney
Fiona Beddall
Rand Cardwell
Jesse Romero
Paige Powers
Arrl Inc
Philip Purser Hallard
John H Cunningham
Maurice J Thompson
Paul Murdin
Sallyann Beresford
Dory Willer
Douglas Preston
Pam Flowers
Edward Humes
Charles Staley
Sylvia Williams Dabney
James Miller
Veronica Roth
Nicolas Bergeron
Malika Grayson
Ron Senyor
Dan Flores
Julietta Suzuki
Oliver Sacks
Dr Tommy John
Lucy Cooke
Rick Trickett
Della Ata Khoury
Richard Drake
Wayne Coffey
Caitlyn Dare
Steven Kerry Brown
Richard W Voelz
Joanne V Hickey
Katharine Mcgee
Jack Disbrow Gunther
Ivy Hope
Collins Easy Learning
Sheri Morehouse
Maha Alkurdi
Bruce W Harris
Vladimir Lossky
Norman Thelwell
Caleb J Tzilkowski
Laurie Notaro
Heather Jacobson
Meg Cabot
Robert A Cutietta
Linda Rosenkrantz
Stella Cottrell
Tim S Grover
Marco Wenisch
Thomas Achatz
Jessica Holsman
Nicola Yoon
Chad Eastham
Michael Blastland
Christine Mari Inzer
Scott Mactavish
Richard Barrett
Rita Golden Gelman
Jared Diamond
Maren Stoffels
Sarah Jacoby
Shmuel Goldberg
Barbara Acello
Trevelyan
Craig Callender
E Ink Utilizer
John H Falk
John Moren
Robert Larrison
Skip Lockwood
Simon A Rego
C M Carney
John J Ratey
Julia Ann Clayton
David Tanis
Sara Gaviria
Douglas P Fry
Lily Raff Mccaulou
Jack Canfield
Kendall Rose
Richard Bullivant
Bryan Litz
John Vince
Mcgraw Hill
Don S Lemons
Mary Pagones
Tom Bass
Michael Chatfield
Samantha De Senna Fernandes
Earl G Williams
Elizabeth Laing Thompson
Mark Young
Jim Supica
Donna Goldberg
Gregory A Kompes
Jasmine Shao
Simon Michael Prior
Matt Mullenix
Deirdre V Lovecky
Db King
Marcia Scheiner
Lianna Marie
Rowena Bennett
Ken Sande
Susan Garcia
Huberta Wiertsema
Eugenia G Kelman
Frederick Jackson Turner
William M Baum
Lily Field
Tyler Trent
Katie Fallon
Janet Evans
Hibiki Yamazaki
Terry Laughlin
Lsat Unplugged
Roger J Davies
Kevin Panetta
Brandy Colbert
Jill Angie
Larry Larsen
Anthony Camera
Sophie D Coe
Sue Elvis
Richard Harding Davis
Matt Baglio
Freya Pickard
Carlos I Calle
David Savedge
Dan Romanchik Kb6nu
Carrie Marie Bratley
Max Lucado
Stian Christophersen
Michael Mewshaw
Matt Price
Robert Moor
Paul Lobo
Pedro Urvi
George Daniel
Ivan Gridin
Natasha Ngan
Kat Davis
Dr Monika Chopra
Trevor Thomas
Niels H Lauersen
James Beard
Elizabeth Dupart
Joyceen S Boyle
Julie Golob
Dvora Meyers
K C Cole
Emily Lowry
Sophie Messager
Shyima Hall
Nicholas Tomalin
Kevin A Morrison
Nedu
John R Mabry
Vincent Chidindu Asogwa
Tibor Rutar
Aaron Reed
Paul A Offit
Ronald T Potter Efron
Katherine D Kinzler
Jess J James
Emt Basic Exam Prep Team
Guy Grieve
Diane Lindsey Reeves
Marc Van Den Bergh
Shelby Hailstone Law
Jeffrey L Kohanek
Eric R Dodge
M E Brines
Buddy Levy
Lynette Noni
Sarah Prager
Sam Priestley
Kaplan Test Prep
Jason Runkel Sperling
Donna R Causey
Helen Webster
Marla Taviano
Kevin Houston
Karen J Rooney
Robert Edward Grant
Shaunti Feldhahn
Martina D Antiochia
Lois Lowry
Mo Gawdat
Shea Ernshaw
Thomas Daniels
Ruthellen Josselson
Law School Admission Council
Peter Bodo
Celeste Headlee
Dianne Maroney
Fmg Publications Special Edition
Joe Baker
Pia Nilsson
Melissa Mullamphy
Heather Balogh Rochfort
Sandra Niche
Mark Stanton
Orangepen Publications
Daniel Prince
Kruti Joshi
Judith Merkle Riley
Bruce Watt
Sheila A Sorrentino
Susan Frederick Gray
Peter J D Adamo
Silvia Dunn
W D Wetherell
Francis Glebas
Declan Lyons
Dave Bosanko
Marisa Peer
Robert D Gibbons
Max Prasac
Jim Warnock
J Maarten Troost
Kathleen Flinn
Roger Marshall
Leslie R Schover
Wilhelm Reich
David Wilber
Matt Racine
Jamie Marich
Maxine A Goldman
Elizabeth May
J R Rain
Mona Bijjani
William Rathje
Jacob Erez
Sterling Test Prep
Michael A Tompkins
Howard E Mccurdy
Mary C Townsend
Mathew Orton
Emily Writes
Warwick Deeping
Dounya Awada
Sonia Shah
Pamela Weintraub
Chris Bonington
Lisa Hopp
Emma Warren
Sarah Ockwell Smith
Lynn Butler Kisber
Jeff Scheetz
Max Lugavere
Creek Stewart
Jennifer Appel
Luc Mehl
Ira K Wolf
Scott Cawthon
Ralph Galeano
Ronit Irshai
Stephen King
John Grehan
Cathy Glass
Jen Howver
Leah Zani
Valerie Poore
Tamara Ferguson
Rebecca Musser
Michelle Travis
Stephen Harrison
David Eagleman
Kathy Woods
Upton Sinclair
Chris Morton
Charles Sanger
Philippe Karl
Lew Freedman
Carole Bouchard
Hecateus Apuliensis
Bryce Carlson
Nick Littlehales
Joel Best
Olivier Doleuze
Cassandra Mack
Bruce Van Brunt
Matthew Warner Osborn
Thomas Deetjen
Caroline Manta
Lee Jackson
Henry Malone
Melanie Anne Phillips
Ian Leslie
C S Lewis
David Nathan Fuller
Elaine Tyler May
Kindle Edition
Craig Martelle
Sammy Franco
Issai Chozanshi
Greg W Prince
Lisa Feldman Barrett
Kerry H Cheever
Nick Tumminello
Helen Zuman
Ashley Eckstein
Joseph Moss
Carol Inskipp
Don Allen Jr
John Kretschmer
Stedman Graham
Erin Macy
Lottie Bildirici
C F Crist
Victoria Honeybourne
Troy Horne
Elena Paige
Jennifer Rose
Albert Jeremiah Beveridge
Dian Olson Belanger
Kyra Phillips
Jimmy Chin
Lee Alan Dugatkin
Gary Lewis
Ransom Riggs
Tanya Hackney
Oprah Winfrey
Nicola S Dorrington
Cecil B Hartley
Clement Salvadori
Tricia Levenseller
James W Anderson
Byron L Reeder
Frank Muir
Jim Kempton
Steve Barrett
Steven W Dulan
Eric P Lane
Richard C Francis
Valliappa Lakshmanan
William L Sullivan
Erin Beaty
Ed Housewright
Robert Garland
Veronica Eden
E W Barton Wright
Cameron Mcwhirter
Steve Guest
Jonathan T Gilliam
Jennifer L Scott
Richard Henry Dana
Ned Feehally
J R Harris
Matthew Marchon
Jessica Howard
Scott Hartshorn
Joseph Correa
Jane Brocket
Gary Mayes
William Ellet
Stacey Rourke
Narain Moorjani
J D Williams
Susan Orlean
Tyler Burt
Graham Norton
Albert Rutherford
Doug Cook
Leslie A Sams
Tey Meadow
Duncan Steel
Jim West
Ken Schwaber
Mike X Cohen
Elmer Keith
Ron Rapoport
Romola Anderson
Winslow Tudor
John M Marzluff
Tony E Adams
Lina K Lapina
Justin Coulson
Hugh Aldersey Williams
Robert Walker
Kelly Rowland
Winky Lewis
Bunmi Laditan
Keith Brewer
Ivar Dedekam
Natasha Daniels
Charu C Aggarwal
Scott Mcmillion
Sarah Berman
Jim Al Khalili
Destiny S Harris
R L Medina
C J Archer
John Flanagan
Meghan Daum
Kacen Callender
Rob Pate
Meriwether Lewis
Fern Schumer Chapman
Carmen Davenport
Tim Freke
Phil Bourque
Bruce Sutherland
Konstantinos Mylonas
Megan Miller
Graham Hancock
Suzanne Young
Denise May Levenick
Dick Hannula
Darcy Lever
Dorothy Canfield Fisher
Martina Mcbride
S M Kingdom
Tom Cunliffe
Martin Davies
Mark Stavish
Kevin Howell
William Wood
Elisabeth Elliot
Bruce Maxwell
Nick Gamis
Tim Glover
Ian Tuhovsky
Light bulbAdvertise smarter! Our strategic ad space ensures maximum exposure. Reserve your spot today!
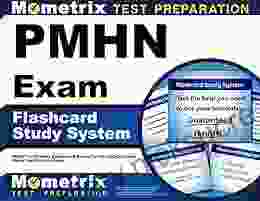

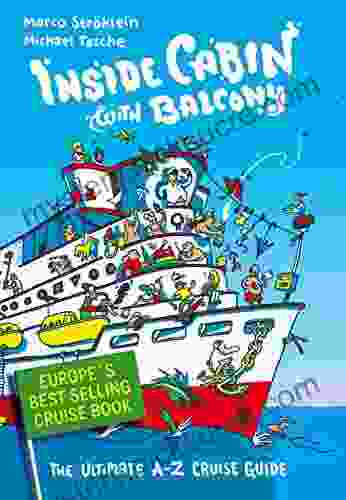

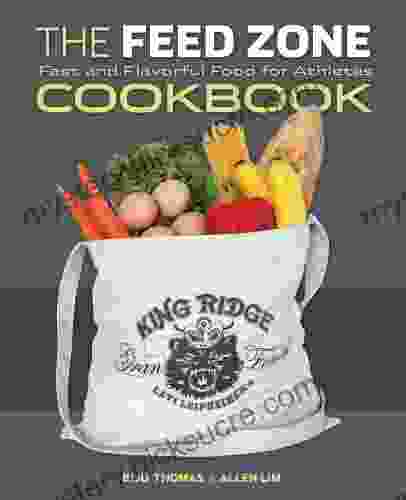

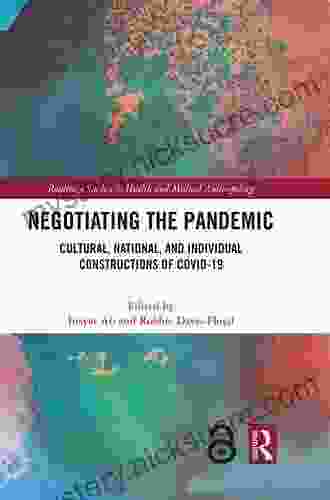

- Shaun NelsonFollow ·19.7k
- Billy FosterFollow ·12.7k
- Oliver FosterFollow ·10.6k
- Edison MitchellFollow ·12.8k
- W. Somerset MaughamFollow ·7.7k
- Felix CarterFollow ·19.5k
- Chandler WardFollow ·15.1k
- Frank ButlerFollow ·18.4k
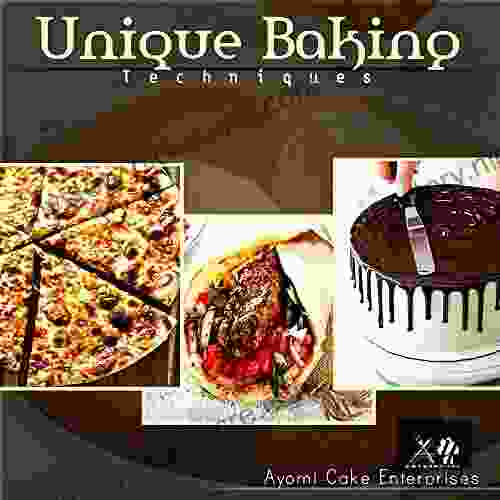

How To Bake In Unique Way: Unleash Your Culinary...
Baking is an art form that transcends the...
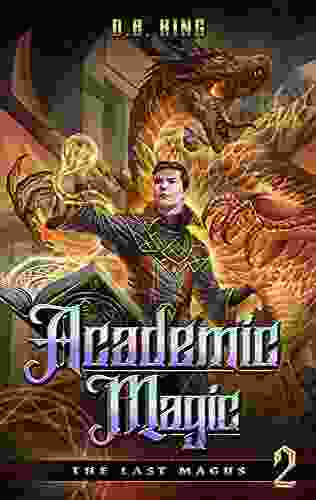

Academic Magic: Unveil the Secrets of The Last Magus
Delve into a Realm of...
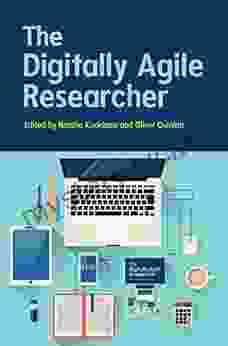

The Digitally Agile Researcher in UK Higher Education:...
In the rapidly...
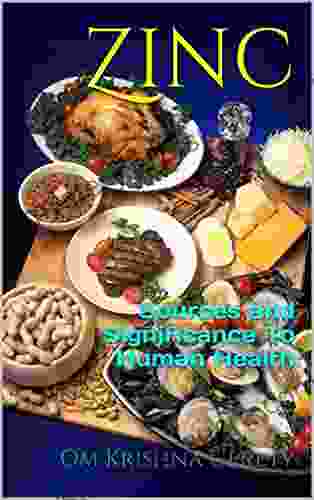

Zinc: Sources And Significance To Human Health
Zinc, an essential trace mineral, plays a...
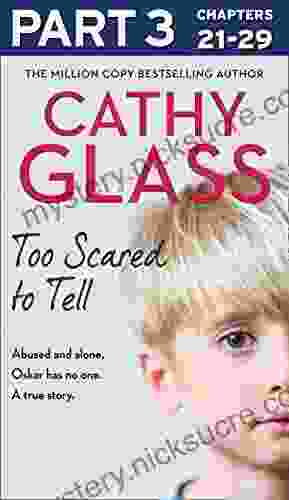

Too Scared to Tell: A Harrowing and Thought-Provoking...
In the realm...
4 out of 5
Language | : | English |
File size | : | 6464 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Word Wise | : | Enabled |
Print length | : | 263 pages |
Lending | : | Enabled |