Linear Algebra: Theory, Intuition, and Code

Linear algebra is a branch of mathematics that deals with vectors, matrices, and linear transformations. It is used in a wide variety of applications, including computer graphics, physics, engineering, and economics.
4.7 out of 5
Language | : | English |
File size | : | 19245 KB |
Screen Reader | : | Supported |
Print length | : | 589 pages |
Lending | : | Enabled |
This article will provide an in-depth exploration of linear algebra, covering its theoretical foundations, intuitive explanations, and practical applications with code examples.
Vectors
A vector is a mathematical object that has both magnitude and direction. It is represented by an arrow, with the length of the arrow representing the magnitude and the direction of the arrow representing the direction.
Vectors can be added, subtracted, and multiplied by scalars. The addition of two vectors is another vector that points in the direction of the sum of the two original vectors. The subtraction of two vectors is another vector that points in the direction of the difference between the two original vectors. The multiplication of a vector by a scalar is another vector that points in the same direction as the original vector, but with a magnitude that is the product of the original magnitude and the scalar.
Matrices
A matrix is a rectangular array of numbers. It is used to represent a linear transformation. A linear transformation is a function that takes a vector as input and produces another vector as output.
Matrices can be added, subtracted, and multiplied by scalars. The addition of two matrices is another matrix that is the sum of the two original matrices. The subtraction of two matrices is another matrix that is the difference between the two original matrices. The multiplication of a matrix by a scalar is another matrix that is the product of the original matrix and the scalar.
Linear Transformations
A linear transformation is a function that takes a vector as input and produces another vector as output. Linear transformations are used to represent a wide variety of operations, including rotations, translations, and scaling.
Linear transformations can be represented by matrices. The matrix that represents a linear transformation is called the transformation matrix.
Eigenvalues and Eigenvectors
An eigenvalue is a scalar that is associated with an eigenvector. An eigenvector is a vector that is not changed by a linear transformation.
Eigenvalues and eigenvectors are used to find the principal axes of a transformation. The principal axes are the directions in which the transformation stretches or shrinks the most.
Applications of Linear Algebra
Linear algebra is used in a wide variety of applications, including:
- Computer graphics
- Physics
- Engineering
- Economics
In computer graphics, linear algebra is used to represent 3D objects and to perform transformations on those objects.
In physics, linear algebra is used to represent forces and to solve equations of motion.
In engineering, linear algebra is used to design structures and to analyze vibrations.
In economics, linear algebra is used to model economic systems and to forecast economic growth.
Linear algebra is a powerful tool that can be used to solve a wide variety of problems. It is a fundamental tool in many fields, including computer graphics, physics, engineering, and economics.
This article has provided an in-depth exploration of linear algebra, covering its theoretical foundations, intuitive explanations, and practical applications with code examples.
Code Examples
The following code examples demonstrate how to use linear algebra to solve a variety of problems.
Example 1: Solving a system of linear equations
python import numpy as np
# Define the coefficient matrix A = np.array([[1, 2], [3, 4]])
# Define the right-hand side vector b = np.array([5, 7])
# Solve the system of linear equations x = np.linalg.solve(A, b)
# Print the solution print(x)
Example 2: Finding the eigenvalues and eigenvectors of a matrix
python import numpy as np
# Define the matrix A = np.array([[1, 2], [3, 4]])
# Find the eigenvalues and eigenvectors eigenvalues, eigenvectors = np.linalg.eig(A)
# Print the eigenvalues and eigenvectors print(eigenvalues) print(eigenvectors)
References
- Linear Algebra Done Right
- MIT OpenCourseWare: Linear Algebra
- Khan Academy: Linear Algebra
4.7 out of 5
Language | : | English |
File size | : | 19245 KB |
Screen Reader | : | Supported |
Print length | : | 589 pages |
Lending | : | Enabled |
Do you want to contribute by writing guest posts on this blog?
Please contact us and send us a resume of previous articles that you have written.
Fiction
Non Fiction
Romance
Mystery
Thriller
SciFi
Fantasy
Horror
Biography
Selfhelp
Business
History
Classics
Poetry
Childrens
Young Adult
Educational
Cooking
Travel
Lifestyle
Spirituality
Health
Fitness
Technology
Science
Arts
Crafts
DIY
Gardening
Petcare
Nicolas Bergeron
Caitlyn Dare
Jonathan T Gilliam
Jennifer Rose
Leslie A Sams
Norman Thelwell
Jesse Romero
Charu C Aggarwal
Lisa Feldman Barrett
Elizabeth Laing Thompson
Shea Ernshaw
Tey Meadow
Sonia Shah
Mary Pagones
Jennifer L Scott
Sophie D Coe
Carlos I Calle
Om Krishna Uprety
Paul Rabinow
Steven Kerry Brown
Shawna Richer
Kelly Rowland
Maxine A Goldman
David Wilber
Nicola S Dorrington
C S Lewis
Lily Field
Kat Davis
Huberta Wiertsema
Cassandra Mack
Dave Rearick
Leah Hazard
James Goi Jr
Sandra Niche
Maha Alkurdi
Joel Best
Kevin Panetta
Chris Bonington
Linda Rosenkrantz
Dounya Awada
Matthew Marchon
Michael Chatfield
Tricia Levenseller
Winky Lewis
Max Lucado
Eric P Lane
Craig Callender
Rob Pate
Stedman Graham
Robert Edward Grant
Jen Howver
Erin Beaty
Chris Pountney
Wyatt Mcspadden
Scarlett V Clark
Carrie Marie Bratley
Matt Racine
Lauren Manoy
Warwick Deeping
Zachery Knowles
Jacob Erez
Karen J Rooney
Db King
Carole Bouchard
Douglas P Fry
Rowena Bennett
Richard C Francis
James Miller
Denise May Levenick
Terry Laughlin
Melanie Anne Phillips
Nicola Yoon
Simon A Rego
Lottie Bildirici
David Savedge
Max Prasac
Bruce Sutherland
Tom Cunliffe
Thomas Carothers
Joseph Moss
Richard Harding Davis
Cameron Mcwhirter
Peterson S
Mark Stavish
Chad Eastham
Dorothy Canfield Fisher
Roger Marshall
Bruce W Harris
Meghan Daum
Lily Raff Mccaulou
Cathy Glass
Arrl Inc
Steve Barrett
Niels H Lauersen
Leon Speroff
Greg W Prince
Tim Glover
Brandy Colbert
Ralph Galeano
Jack Disbrow Gunther
Richard W Voelz
Jill Angie
Thomas Daniels
The Atavist
John R Mabry
Charles Staley
Joe Baker
Bruce Van Brunt
W D Wetherell
Joseph Correa
William L Sullivan
Robert Walker
Jennifer Appel
Cal Newport
Elisabeth Elliot
Ira K Wolf
Jessica Holsman
John H Cunningham
Stephen Harrison
Christine Mari Inzer
Anthony Camera
John Kretschmer
J Maarten Troost
Tony E Adams
Scott Hartshorn
Emma Warren
Martha Finley
J R Rain
Sara Gaviria
Luc Mehl
Kyra Phillips
James Beard
Lynette Noni
Buddy Levy
Howard E Mccurdy
Vincent Chidindu Asogwa
Elizabeth Dupart
Sarah Ockwell Smith
Natasha Ngan
Phil Williams
Kevin Howell
Ronald T Potter Efron
Gregory A Kompes
K C Cole
Tibor Rutar
Larry Larsen
M E Brines
Helen Zuman
Elizabeth Anne Wood
John Jamieson
Paul Lobo
Jeff Belanger
Sarah Berman
Jim Supica
Megan Miller
Albert Rutherford
William M Baum
Donna R Causey
William Rathje
Frank Muir
Byron L Reeder
Shaunti Feldhahn
Maria Van Noord
Mathew Orton
Lianna Marie
Narain Moorjani
Michael Lear Hynson
Pedro Urvi
John Flanagan
Melissa Mullamphy
Dianne Maroney
Jeffrey L Kohanek
Janet Evans
Marisa Peer
Konstantinos Mylonas
Meriwether Lewis
Kathy Woods
Oliver Sacks
Judith Merkle Riley
Joanne Kimes
Sallyann Beresford
L W Jacobs
Nicholas Tomalin
Vivian Foster
Elaine Tyler May
Guy Grieve
Shmuel Goldberg
Jim West
Tom Bass
John M Marzluff
Leah Zani
C F Crist
Cornelia Pelzer Elwood
R Scott Thornton
Bunmi Laditan
Robert Moor
Doug Cook
Dick Hannula
Ransom Riggs
Malika Grayson
Tyler Trent
Jim Wiese
Paul Murdin
John Grehan
Ron Rapoport
Fern Schumer Chapman
Stella Cottrell
Collins Easy Learning
Katherine D Kinzler
Steven W Dulan
Silvia Dunn
Pia Nilsson
R L Medina
Sarah Baker
Elena Paige
Jack Canfield
Sheila A Sorrentino
Keith Brewer
Celeste Headlee
Daniel Prince
Dennis Adler
Kevin A Morrison
Jasmine Shao
Trevelyan
Robert Larrison
Bryce Carlson
Aaron Reed
John H Falk
Kathleen Flinn
Winslow Tudor
Justin Coulson
Veronica Roth
Caleb J Tzilkowski
Meg Cabot
William Wood
Eric R Dodge
Sherri L Jackson
Wilhelm Reich
Robert D Gibbons
Jimmy Chin
Sheri Morehouse
Susan Garcia
Michael Tan
Diane Lindsey Reeves
Douglas Preston
Hecateus Apuliensis
Pamela Weintraub
Martin Davies
Marc Bona
Dian Olson Belanger
Graham Hancock
Nick Tumminello
Don Allen Jr
Shelby Hailstone Law
Samantha De Senna Fernandes
Nigel Cawthorne
James W Anderson
David Eagleman
Tim S Grover
Declan Lyons
Carol Inskipp
Fmg Publications Special Edition
Bryan Litz
Kaplan Test Prep
Troy Horne
Emily Writes
Dr Monika Chopra
Mark Young
Dvora Meyers
Dan Romanchik Kb6nu
Jeff Scheetz
Rebecca Musser
Maurice J Thompson
Kruti Joshi
Issai Chozanshi
Richard Drake
Marco Wenisch
William Ellet
Suzanne Young
Ronit Irshai
Matt Price
Nick Gamis
Tanya Hackney
Rita Golden Gelman
Shyima Hall
Francis Glebas
Mona Bijjani
Mcgraw Hill
Lee Jackson
Nikki Carroll
Helen Webster
Marcia Scheiner
David Nathan Fuller
Ivan Gridin
Duncan Steel
Philip Purser Hallard
Richard Bullivant
Elizabeth May
Jim Al Khalili
Della Ata Khoury
Skip Lockwood
Ivar Dedekam
Clement Salvadori
Ivy Hope
Ashley Eckstein
Maren Stoffels
Lewis Kirkham
Trevor Thomas
Neville Goddard
Edward Humes
Henry Malone
Pam Flowers
Elmer Keith
Bruce Watt
William Ian Miller
Sammy Franco
Kevin Houston
John Moren
Lynn Butler Kisber
J D Williams
Charles Sanger
Richard Barrett
Stephen King
Veronica Eden
Matt Mullenix
Martina Mcbride
Marla Taviano
Scott Mcmillion
Michael D Alessio
Thomas Deetjen
Kacen Callender
Emily Lowry
Victoria Honeybourne
Pat Rigsby
Mo Gawdat
Kindle Edition
Julie Golob
Michael Gurian
Earl G Williams
Tyler Burt
Ned Feehally
Orangepen Publications
Max Lugavere
Michael Blastland
Paige Powers
Julia Ann Clayton
Erin Macy
Philippe Karl
Hibiki Yamazaki
Valliappa Lakshmanan
Scott Mactavish
Oprah Winfrey
Jane Brocket
Mark Stanton
Peter J D Adamo
Thomas Achatz
Mark Hansen
Sarah Prager
Law School Admission Council
Dory Willer
Carmen Davenport
Nawuth Keat
Katharine Mcgee
Robert Garland
Sylvia Williams Dabney
Lois Lowry
Eugenia G Kelman
Tim Freke
Matthew Warner Osborn
David Cannon
Ian Leslie
Theodora Papatheodorou
Leslie R Schover
Rand Cardwell
Susan Orlean
E W Barton Wright
Lucy Cooke
Jessica Howard
Charles Salzberg
Heather Jacobson
Dan Flores
Laurie Notaro
Stacey Rourke
Joanne V Hickey
Creek Stewart
Fiona Beddall
Ed Housewright
Julietta Suzuki
Deirdre V Lovecky
George Daniel
Ian Tuhovsky
Heather Balogh Rochfort
Lisa Hopp
Nedu
Richard Henry Dana
Lee Alan Dugatkin
Jim Warnock
Robert A Cutietta
John Burroughs
Craig Martelle
Ken Sande
Jason Runkel Sperling
Healthfit Publishing
Jared Diamond
Dr Tommy John
John J Ratey
Frederick Jackson Turner
Romola Anderson
S M Kingdom
Tina Schindler
Joyceen S Boyle
Sarah Jacoby
Albert Jeremiah Beveridge
Dave Bosanko
Ashley Christensen
Chris Morton
E Ink Utilizer
Susan Frederick Gray
Bruce Maxwell
Lina K Lapina
Scott Cawthon
Valerie Poore
Jim Kempton
Roger J Davies
John Vince
Vladimir Lossky
Kerry H Cheever
Kendall Rose
Cecil B Hartley
David Martin
Nick Littlehales
Simon Michael Prior
Matt Baglio
Sam Priestley
Don S Lemons
Ruthellen Josselson
David Tanis
C J Archer
Hugh Aldersey Williams
Sterling Test Prep
Carrie Hope Fletcher
Graham Norton
Barbara Acello
Michael Mewshaw
Lingo Mastery
Dina Nayeri
Gary Lewis
Sue Elvis
J R Harris
Sophie Messager
Jess J James
Ken Schwaber
Gary Mayes
Marc Van Den Bergh
Rick Trickett
Upton Sinclair
Mike X Cohen
Martina D Antiochia
Tamara Ferguson
Emt Basic Exam Prep Team
Lsat Unplugged
Phil Bourque
Freya Pickard
Wayne Coffey
Mary C Townsend
Steve Guest
Stian Christophersen
Olivier Doleuze
Jamie Marich
Paul A Offit
Caroline Manta
Katie Fallon
Michelle Travis
Destiny S Harris
Donna Goldberg
Michael A Tompkins
Natasha Daniels
Lew Freedman
Peter Bodo
Darcy Lever
Melissa A Priblo Chapman
C M Carney
Ron Senyor
Light bulbAdvertise smarter! Our strategic ad space ensures maximum exposure. Reserve your spot today!
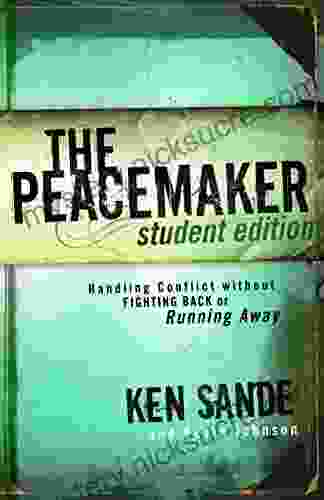

- Lawrence BellFollow ·2.5k
- Darius CoxFollow ·11.2k
- Ricky BellFollow ·6.1k
- Ken SimmonsFollow ·4.6k
- Javier BellFollow ·18.5k
- Ian McEwanFollow ·6.5k
- Luke BlairFollow ·10.2k
- Henry David ThoreauFollow ·10.8k
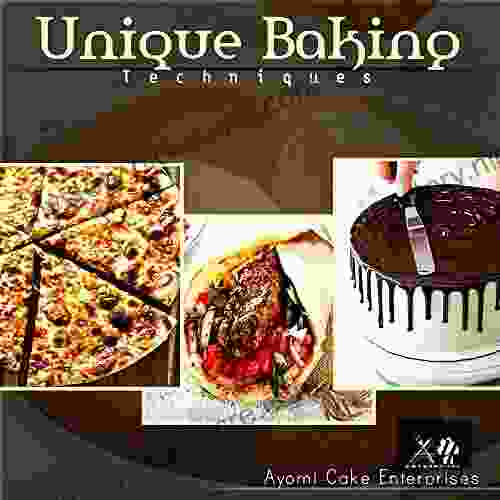

How To Bake In Unique Way: Unleash Your Culinary...
Baking is an art form that transcends the...
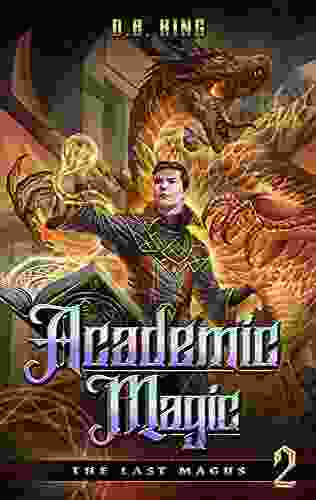

Academic Magic: Unveil the Secrets of The Last Magus
Delve into a Realm of...
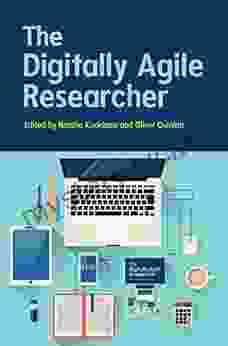

The Digitally Agile Researcher in UK Higher Education:...
In the rapidly...
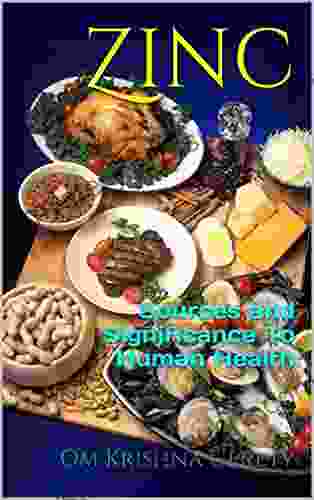

Zinc: Sources And Significance To Human Health
Zinc, an essential trace mineral, plays a...
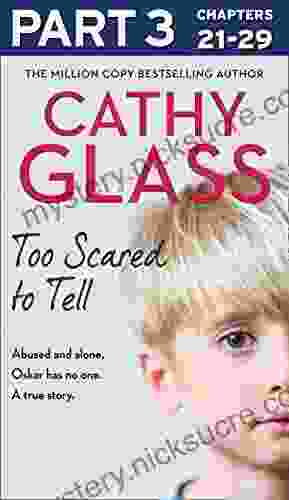

Too Scared to Tell: A Harrowing and Thought-Provoking...
In the realm...
4.7 out of 5
Language | : | English |
File size | : | 19245 KB |
Screen Reader | : | Supported |
Print length | : | 589 pages |
Lending | : | Enabled |